I use Rails both professionally and personally for more than a decade now. Through the years, my usage of Rails also evolved together with new concepts and trends in web development. Here are some of the personal changes I am applying moving forwards on how I work with the framework.
Authentication using JWT
JSON Web Tokens (JWT) provides a way to effectively secure API endpoints. As web applications move more to the front-end with the popularity of Javascript frameworks like React and Angular, JWT ensures that the connection between the front-end and the back-end remains secure.
However, I don’t believe that we should reinvent the wheel, especially when it comes to authentication. Thus I will still continue to use the devise gem. This library has been a staple in Rails applications for many years now and as a result has already gone through a lot of patching and bug fixes.
There’s also a gem called devise-jwt that makes it easy to integrate devise authentication using JWT, and I am using this library for present and future projects.
What this gem does is that after a user signs in, it automatically adds a JWT token to the Authorization header. This token can then be used by the front-end code to submit requests back to the server. The server checks the token and validates if the user has access to the endpoint or resource. Using the gem, this is being done automatically, and that user is available through the current_user variable.
You can still use the (default) session authentication as well, so you can have both a web application and a front-end client application (like a mobile app) using the same authentication mechanism.
dry-rb
Rails is an opinionated framework. This means it has its own way of doing things, and by following them it can make your work simpler and faster. These methods utilize the full features of the framework and lets the framework do the heavy lifting for you. Some people do not like this approach as it makes things less transparent and “magical”.
While Rails is opinionated, it is not restrictive. You can ignore built-in features or build your own without having the need to abandon the framework altogether.
There are several libraries that enable you to work outside the Rails conventions. Some of the most popular ones are Trailblazer and dry-rb. Moving forward, I intend to use dry-rb more as I found that their modular approach to libraries is a great way to implement projects.
Here are some of the concepts that I found useful in dry-rb:
Transactions and Operations
Using the transaction concept allows us to think of a feature in terms of steps. This in turn enables us to break down a feature into smaller parts. It also allows for easier debugging and refactoring of code as we can create steps that essentially just perform one specific task of the whole.
As an example, let’s say we want to create an event and send an email to its participants. In a transaction, we can break this down into three steps: checking the event form parameters, creating the record, and then sending the emails.
require "dry/transaction"
class CreateEvent
include Dry::Transaction
step :validate_parameters
step :create_event
step :send_email, with: 'event.operations.send_email'
private
def validate_parameters(params)
# returns Success(valid_params) or Failure(errors)
end
def create_event(params)
# returns Success(event)
end
end
You can see that the validate_parameters and create_event methods are simply private methods of the transaction class. The send_email method however is not present in the class itself but is invoked as an external operation. For this example, we can have an operation class called Event::Operations::SendEmail.
Using operation classes allows us to create reusable functions. These operations can be called on any other transaction (or any other code really). In addition to being reusable and modular, it is also easy to test as each operation performs only one specific function.
Monads
Notice that the return values of the methods are Success() and Failure(). These are called monads and they help structure your responses so that methods communicate with each other more effectively. The value I get from this is it forces me to think of failure scenarios in the code. I can then handle success and failure conditions effectively without resorting to complex logic or nested if-else conditions.
Validations
dry-rb also has a library called dry-validation. Using this library you can define your own custom schema and validation rules. In Rails, the default schema and validation is usually tied to your database models. dry-validation provides the same feature that you can use on any code in your application.
It also enables you to define types in each of the fields in your schema. This can simplify your code as you are guaranteed that each field has the correct type. This also reduces potential bugs in your application.
Here is an example found in their official documentation. It describes how to use dry-validation in setting up the schema and the validation for a new User record:
class NewUserContract < Dry::Validation::Contract
params do
required(:email).filled(:string)
required(:age).value(:integer)
end
rule(:email) do
unless /\A[\w+-.]+@[a-z\d-]+(.[a-z\d-]+)*.[a-z]+\z/i.match?(value)
key.failure('has invalid format')
end
end
rule(:age) do
key.failure('must be greater than 18') if value < 18
end
end
These are just some of the ways you can use dry-rb. There are plenty more gems and libraries that they provide that can be of use in your Ruby application.
ERB instead of haml
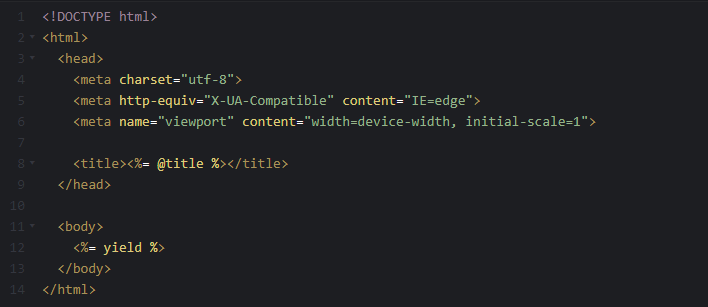
As I delve more into front-end development, I find myself using plain old HTML files instead of templating languages like haml or slim. When I started using Rails many years ago I hated using ERB for views as it was too verbose. Now I find myself going full circle and going back to ERB views.
All web developers should learn how to write plain old HTML code. It is important for us to know how it works behind the web application frameworks we are using. Knowing the fundamentals of HTML and CSS (and some Javascript) will already get you a long way when creating a website.
Documentation and tutorials relating to web development almost always show HTML instead of a templating system. When you are learning new technologies like React or exploring a CSS framework, the code examples and snippets are all plain HTML files. Thus it is easier to incorporate them in your application than having to convert them first to a template language.
When working with JSX for example, you will be writing “HTML-like” code in your React components all the time. Knowing how HTML is structured and how CSS affects them is a necessary part of designing front-end components.
Still in Rails
I still find it a lot of fun to work in Rails. It still enables you to be productive and create web applications very fast. The framework is evolving together with the web development landscape as we can see in the release of Rails 6. I hope these personal changes also help you think of ways on how to use Rails more effectively.